Thank you for your input,
I already had that in my code. What I managed to observe is that somehow when I resize the physical window the following class instances do resize as well: QMainWindow, QWidget on top of which the TCanvas is based and here comes a tricky part. The TPad inside of that TCanvas changes it’s size just as expected but the TCanvas itself does not
. I tried inspecting that and all I managed to find out was that somehow a new instance of TCanvas was created ?
I am just leaving a small snippet of my code(maybe that will be enough to showcase the problem until I come up with a proper example code):
class TCanvasContainer: public QWidget{
//My main pointer that (was supposed) to hold the histograms
TCanvas* m_canvas = nullptr;
...
}
//CTOR:
int wid = gVirtualX->AddWindow((ULong_t)winId(), width(), height());
m_canvas = new TCanvas("Root Canvas", width(), height(), wid);
// I handle resizing in the resizeEvent() method within the MainWindow class.
virtual void resizeEvent( QResizeEvent * event) override;
// It basically takes the size of the window and passes it further on to the class with the m_canvas where I attempt the following:
void TCanvasContainer::ResizeCanvas(const QSize & p_size) {
std::cout << "m_canvas size: " << m_canvas->GetWw() << ", "
<< m_canvas->GetWh() << std::endl;
std::cout << "MainWindow size: " << p_size.width() << "," << p_size.height() << std::endl;
m_canvas->SetCanvasSize(p_size.width(), p_size.height());
//retrieving the TPad from the m_canvas:
auto pad = static_cast<TPad *>(m_canvas->cd());
std::cout << "TPad size: " << pad->GetWw() << ", " << pad->GetWh() << std::endl;
//retrieving the TCanvas back
auto canv = pad->GetCanvas();
//For some reason this one resizes just fine...
std::cout << "canv size: " << canv->GetWw() << ", " << canv->GetWh() << std::endl;
getEmbeddedCanvas()->displayAddressOfCanvas();
std::cout << "&canv: " << &canv << ", canv: " << canv << std::endl;
gPad->SetCanvasSize(p_size.width(), p_size.height());
pad->SetCanvasSize(p_size.width(), p_size.height());
m_canvas->Update();
}
What gets displayed to the console when I resize the window:
As you can tell the canv resizes just fine and the m_canvas stays the same (except for these moments when it changes for a split second and goes back to the original value).
When It comes to how the actual window behaves:
When resizing it seems as if the actual TCanvas received the singal telling it to resize and the actual plot rescales for a split second and then immediately resets itself back to the original form. (size of the plot stays the same just like in the original post)
That’s the way the plot looks like for that split second:
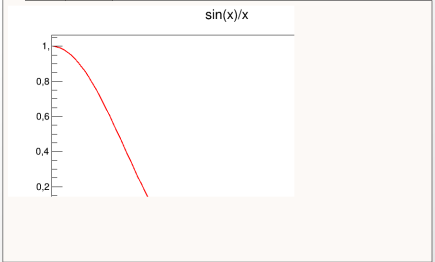
Edit:
I’m guessing blindly that maybe somehow it has to do something with TCanvasImp?
Edit2:
I have spent some extra time with the code and I managed to notice that when constructing the m_canvas in this way (without the Window ID):
m_canvas = new TCanvas("Root Canvas", "test", width(), height());
A new window pops up instead of the previously build in TCanvas. And interestingly all the dependencies are still here - resizing the Main QT window causes the TCanvas within the second window to rescale/resize (it makes it so that it does not fit within the window anymore at some point)